개요
- .append()는 선택한 요소의 내용의 끝에 콘텐트를 추가합니다.
문법
.append( content [, content ] )
예제 1
- 순서 없는 목록 마지막에 Dolor를 추가합니다.
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>jQuery</title>
<style>
body {
line-height: 2;
font-family: sans-serif;
font-size: 20px;
}
</style>
<script src="//code.jquery.com/jquery-3.3.1.min.js"></script>
<script>
$( document ).ready( function() {
$( 'ul' ).append( '<li>Dolor</li>' );
} );
</script>
</head>
<body>
<ul>
<li>Lorem</li>
<li>Ipsum</li>
</ul>
</body>
</html>
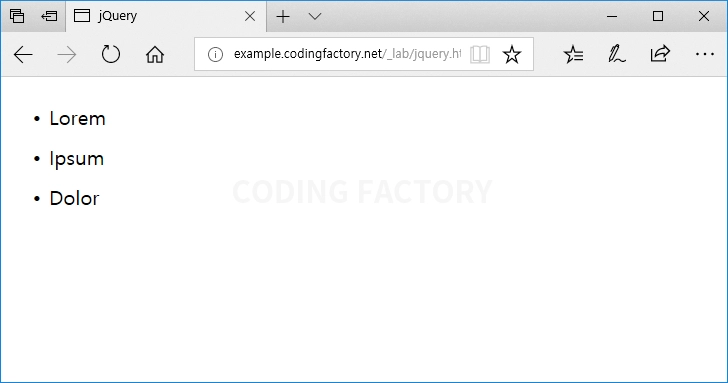
예제 2
- strong 요소를 p 요소의 내용의 끝으로 이동시킵니다.
<!doctype html>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>jQuery</title>
<style>
body {
line-height: 2;
font-family: sans-serif;
font-size: 20px;
}
</style>
<script src="//code.jquery.com/jquery-3.3.1.min.js"></script>
<script>
$( document ).ready( function() {
$( 'p' ).append( $( 'strong' ) );
} );
</script>
</head>
<body>
<p>abc</p>
<strong>XYZ</strong>
</body>
</html>
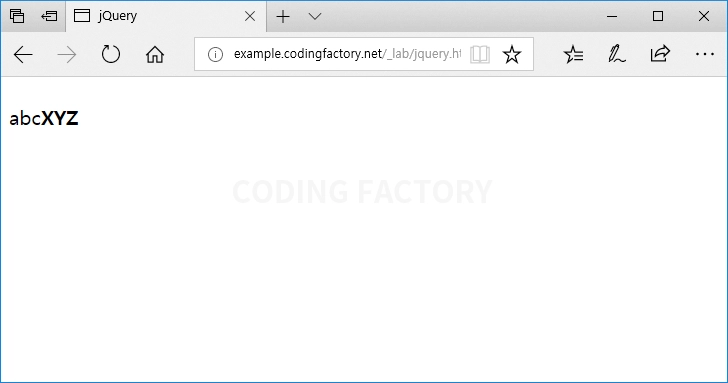